How to connect Strapi to MongoDB Atlas and Docker
Last updated
In this article, I'm going to explain how to set up a Strapi project that uses MongoDB as a database. To run the project locally we'll connect Strapi to MongoDB on Docker and in production, we'll connect our Strapi project to MongoDB Atlas.
As in previous Strapi articles, I've created a basic Strapi project that exposes an API to manage books and authors. It's a very simple project but will be enough to show you how to connect to different MongoDB instances locally and in production. You can download or clone it from this repo.
If you're not familiar with Strapi yet, check out my previous article in which I explain some basic concepts and how it can save you time building APIs.
Setting up a Strapi project with MongoDB running on Docker
To bootstrap our Strapi project, we need a MongoDB database to connect to. Instead of installing MongoDB I like to run it as a Docker container. That way, I do not have a MongoDB service running all the time and I only start it whenever I'm going to work on my Strapi project.
You need to have Docker Desktop installed. You can download it here
Start MongoDB locally with Docker
The first thing we need to do is create a volume in which our MongoDB data will be stored. We just need to run docker volume create mongoData
. We'll indicate our MongoDB container to use this volume every time it starts so we don't lose our data after we shut down our container.
To start the MongoDB container we need to run docker run --rm -d -p 27017:27017/tcp -v mongoData:/data/db mongo:latest
. Notice that we're indicating the port and the volume. Once executed, you'll see something like this:
Just remember to run the same docker run ...
command every time you want to work on your Strapi project.
Create a Strapi MongoDB project
To start a Strapi project that connects to MongoDB you just need to run npx create-strapi-app my-app
(or yarn create strapi-app my-app
) and select the following options:
- Choose your installation type Custom (manual settings)
- Choose your default database client mongo
- Database name: api-books
- Host: 127.0.0.1
- +srv connection: false
- Port (It will be ignored if you enable +srv): 27017
- Username: (empty)
- Password: (empty)
- Authentication database (Maybe "admin" or blank): admin
- Enable SSL connection: (y/N) N
After installing all dependencies you'll have a Strapi project connected to your local MongoDB running on Docker.
Notice that in config/database.js
all the database connection details are loaded from environment variables and, by default (if nothing is provided) it's using the values we indicated when we started the project.
// File: config/database.js
module.exports = ({ env }) => ({
defaultConnection: 'default',
connections: {
default: {
connector: 'mongoose',
settings: {
host: env('DATABASE_HOST', '127.0.0.1'),
srv: env.bool('DATABASE_SRV', false),
port: env.int('DATABASE_PORT', 27017),
database: env('DATABASE_NAME', 'api-books'),
username: env('DATABASE_USERNAME', null),
password: env('DATABASE_PASSWORD', null),
},
options: {
authenticationDatabase: env('AUTHENTICATION_DATABASE', 'admin'),
ssl: env.bool('DATABASE_SSL', false),
},
},
},
})
This means that in production or other environments we can override the default values by creating the correspondent environment variables.
Connect Strapi to MongoDB Atlas in production
MongoDB Atlas offers MongoDB clusters that run on AWS, Google Cloud, or Azure, but you can easily manage them from within the MongoDB website. They are easy to use and the cost is not very high even having a free tier. You can create your Mongo Atlas account here and create your first database cluster.
Create a new user in MongoDB Atlas
You'll need a database user and password to connect our Strapi application to MongoDB Atlas. To create it, log in to your MongoDB Atlas account and go to your cluster, and click on "Database Access" > "Add new database user":
Enter a username and a strong password. Additionally, you can configure the different permissions and roles.
Important! For this example, I've selected read and write access to all databases and collections but you should spend some time configuring your permissions accurately.
Get connection details to MongoDB Atlas
Once we have our user, we need the database cluster connection details. To find them, log in to your MongoDB Atlas account and go to your cluster, click on "Connect" > "Connect your application" and select Node.js. Do not check the "Include full driver code example" checkbox and copy the connection string, which will be pretty similar to this:
mongodb+srv://<username>:<password>@YOUR_CLUSTER_NAME.mongodb.net/<dbname>?retryWrites=true&w=majority
You'll have to replace the username, password, and dbname from the connection string with your own details.
Environment variables to connect Strapi to MongoDB Atlas
As mentioned earlier, we can override the default values in config/database.js
by setting up the correspondent environment variables. To connect Strapi to MongoDB Atlas you'll need to create the following environment variables:
- DATABASE_HOST: This is the part of the connection string we just got from Mongo Atlas after the '@' symbol. The format should be something like this: YOUR_CLUSTER_NAME.mongodb.net/
?retryWrites=true - DATABASE_NAME: The database name you chose in Mongo Atlas.
- DATABASE_USERNAME: The username you created in Mongo Atlas.
- DATABASE_PASSWORD: The user's password
- DATABASE_SRV: true
- DATABASE_SSL: true
You can check out my previous article about how to deploy Strapi applications to production in which you can see how to create the environment variables in DigitalOcean App Platform.
If you are managing your own server and have access to it via SSH, you can create the environment variables running
$ export DATABASE_HOST=YOUR_CLUSTER_NAME.mongodb.net/<dbname>?retryWrites=true
If everything is ok, your Strapi application will be able to connect to MongoDB Atlas 🤘
I hope this helps you setup your Strapi project locally and in production. If you have any questions, feel free to contact me or send me a message on Twitter.
Happy coding!
If you enjoyed this article consider sharing it on social media or buying me a coffee ✌️
Oh! and don't forget to follow me on Twitter where I share tons of dev tips 🤙
Other articles that might help you
my projects
Apart from writing articles in this blog, I spent most of my time working on my personal projects.
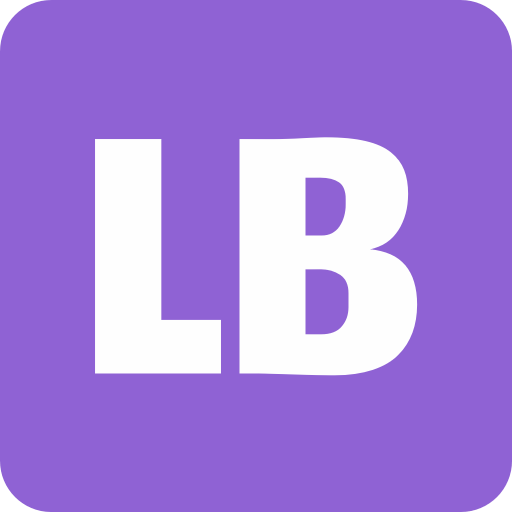
theLIFEBOARD.app
theLIFEBOARD is a weekly planner that helps people achieve their goals, create new habits and avoid burnout. It encourages you to plan and review each week so you can easily identify ways to improve your productivity while keeping track of your progress.
Sign up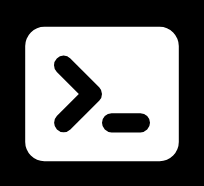
SolidityTips.com
I'm very interested in blockchain, smart contracts and all the possiblilities chains like Ethereum can bring to the web. SolidityTips is a blog in which I share everything I learn about Solidity and Web3 development.
Check it out if you want to learn Solidity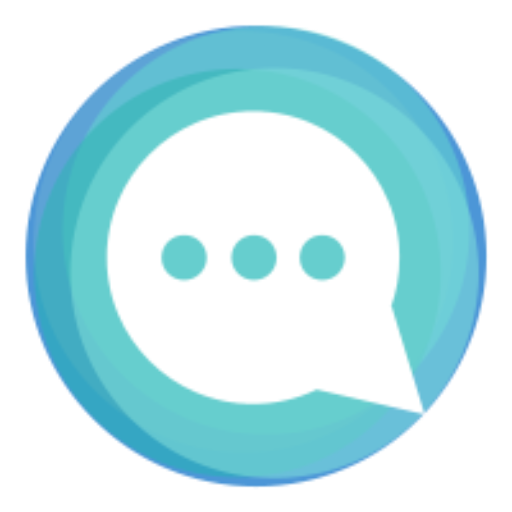
Quicktalks.io
Quicktalks is a place where indie hackers, makers, creators and entrepreneurs share their knowledge, ideas, lessons learned, failures and tactics they use to build successfull online products and businesses. It'll contain recorded short interviews with indie makers.
Message me to be part of it