Two simple tips to improve Vue.js performance and SEO
Last updated
I really like building websites with Vue.js but one of the downsides is the performance is not lightning-fast and SEO is not great. But in order to solve or improve those, there are two things I always do: pre-render all pages I can and use vue-meta to include the correspondent meta-tags (including open-graph) in each page of the application.
If you want to learn how to improve the performance and SEO of your Vue applications, just keep reading 😊
Pre-rendering Vue.js pages
Install the pre-render plugin with vue add prerender-spa
. The installation process will take a few minutes as it'll have to install Chromium, which will be used to "visit" your application pages and capture the generated HTML of each page you want to pre-render.
After the plugin is installed, you'll have to follow a 4 step configuration:
-
1 Which routes to pre-render? -> Just enter the routes you want to pre-render separated by commas, for example: /,/contact,/about
-
2 Use a render event to trigger the snapshot? -> This indicates if you want to wait for the browser to wait for a specific event to capture the page's HTML or just wait for the generic DOMContentLoaded event. Just use the default option which will add a specific event named x-app-rendered in your main.js mounted() hook.
-
3 Use a headless browser to render the application? (recommended) -> If you select no, the plugin will actually open a Chrome tab/window to visit and pre-render your selected routes.
-
4 Only use prerendering for production builds? (recommended) -> Use the default unless you want to pre-render also in development,
You'll be able to find and amend the configuration of the pre-render plugin in the vue.config.js
file.
Now if you run npm run build
the result in your dist
folder will contain the HTML files of the routes you selected. And with this simple change you'll be able to see improvements in performance like these:
IMAGES COMPARE PERFORMANCE
That takes care of pre-rendering, now let's add vue-meta to improve our Vue.js application SEO.
Meta tags in Vue.js
To install the plugin, just run npm i vue-meta
. Then modify the application main.js
file, to import vue-meta and use it as shown below:
// File main.js
import Vue from 'vue'
import App from './App.vue'
import store from './store'
import router from './router'
// imports Vue-meta plugin
import VueMeta from 'vue-meta'
import '@/assets/css/tailwind.css'
Vue.config.productionTip = false
// adds vue-meta to Vue app
Vue.use(VueMeta, {
// optional pluginOptions
refreshOnceOnNavigation: true,
})
// Creates Vue application
new Vue({
router,
store,
render: (h) => h(App),
mounted: () => document.dispatchEvent(new Event('x-app-rendered')),
}).$mount('#app')
The next step is to add in the App.vue
file a base configuration with the title and the common meta tags for all pages, like viewport and description. This has to be included inside the script tag as an object named metaInfo or as a function that returns an object.
You can use the example below which includes meta tags for title, description, and viewport:
// File App.vue
<script>
export default {
// ....
metaInfo() {
return {
// if no subcomponents specify a metaInfo.title, this title will be used
title: 'MyAwesomeApp | Super awesome app built by me',
// all titles will be injected into this template
titleTemplate: '%s | MyAwesomeApp.com',
// Define common meta tags here.
meta: [
{ 'http-equiv': 'Content-Type', content: 'text/html; charset=utf-8' },
{ name: 'viewport', content: 'width=device-width, initial-scale=1' },
{
name: 'description',
content:
'The app built by me that is simply awesome',
},
],
}
},
}
</script>
Then on each page, you'll have to enter the specific meta tags for title, canonical, and OpenGraph (used when you share a link to your site on Facebook/Twitter/etc). See below an example for the contact page
export default {
// ....
metaInfo() {
return {
// title will be injected into parent titleTemplate
title: 'Contact us', // ⚠️ this will generate -> "Contact us | MyAwesomeApp.com"
// specific canonical link for the page
link: [{ rel: 'canonical', href: 'https://MyAwesomeApp.com/contact' }],
// Define page specific openGraph tags
meta: [
// OpenGraph data (Most widely used)
{
property: 'og:title',
content: 'Contact us | MyAwesomeApp',
},
{ property: 'og:site_name', content: 'MyAwesomeApp.com' },
// The list of types is available here: http://ogp.me/#types
{ property: 'og:type', content: 'website' },
// Should the the same as your canonical link, see above.
{ property: 'og:url', content: 'https://MyAwesomeApp.com/contact' },
{
property: 'og:image',
content: 'https://MyAwesomeApp.com/img/logo.png',
},
// Often the same as your meta description, but not always.
{
property: 'og:description',
content: 'Send us a message - MyAwesomeApp',
},
// Twitter card
{ name: 'twitter:card', content: 'summary' },
{ name: 'twitter:site', content: 'https://MyAwesomeApp.com/contact' },
{ name: 'twitter:title', content: 'Contact us |MyAwesomeApp' },
{
name: 'twitter:description',
content: 'Send us a message - MyAwesomeApp',
},
// Your twitter handle, if you have one.
{ name: 'twitter:creator', content: '@MyAwesomeApp' },
{
name: 'twitter:image:src',
content: 'https://MyAwesomeApp.com/img/logo.png',
},
// Google / Schema.org markup:
{ itemprop: 'name', content: 'Contact us | MyAwesomeApp' },
{
itemprop: 'description',
content: 'Send us a message - MyAwesomeApp',
},
{
itemprop: 'image',
content: 'https://MyAwesomeApp.com/img/logo.png',
},
],
}
},
}
Note: make sure that you replace your domain, app name, description, image URL, Twitter account, etc with your application's ones.
Conclusion
With these two simple steps, you'll bump up your Vue.js application performance and SEO. To test your application performance you can use Google's Lighthouse and to validate your OpenGraph meta tags you can use OpenGraph.xyz.
If you find this article useful, consider buying me a coffee using the link below or sharing it on social media!
Happy coding!
If you enjoyed this article consider sharing it on social media or buying me a coffee ✌️
Oh! and don't forget to follow me on Twitter where I share tons of dev tips 🤙
Other articles that might help you
my projects
Apart from writing articles in this blog, I spent most of my time working on my personal projects.
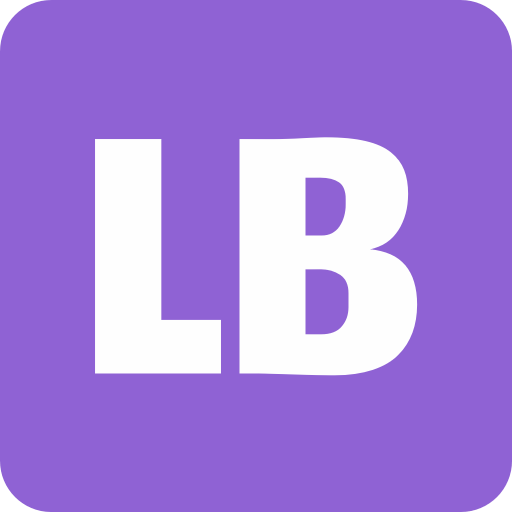
theLIFEBOARD.app
theLIFEBOARD is a weekly planner that helps people achieve their goals, create new habits and avoid burnout. It encourages you to plan and review each week so you can easily identify ways to improve your productivity while keeping track of your progress.
Sign up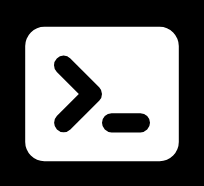
SolidityTips.com
I'm very interested in blockchain, smart contracts and all the possiblilities chains like Ethereum can bring to the web. SolidityTips is a blog in which I share everything I learn about Solidity and Web3 development.
Check it out if you want to learn Solidity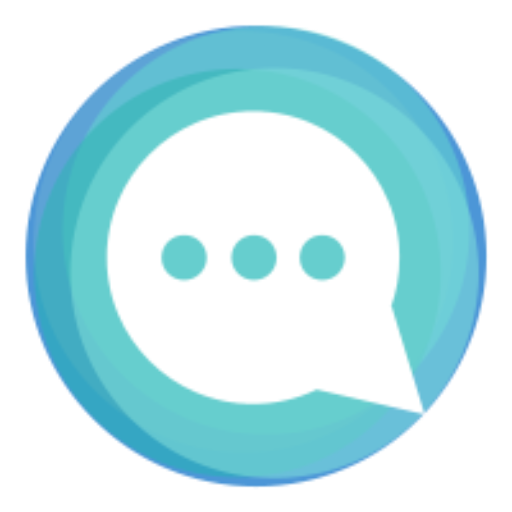
Quicktalks.io
Quicktalks is a place where indie hackers, makers, creators and entrepreneurs share their knowledge, ideas, lessons learned, failures and tactics they use to build successfull online products and businesses. It'll contain recorded short interviews with indie makers.
Message me to be part of it